模拟
简介
使用 Playwright,您可以在任何浏览器上测试您的应用程序,并模拟真实设备,例如手机或平板电脑。只需配置您想要模拟的设备,Playwright 将模拟浏览器行为,例如 "userAgent"
、"screenSize"
、"viewport"
以及是否启用 "hasTouch"
。您还可以为所有测试或特定测试模拟 "geolocation"
、"locale"
和 "timezone"
,以及设置 "permissions"
以显示通知或更改 "colorScheme"
。
设备
Playwright 附带一个设备参数注册表,使用 playwright.devices 用于选定的桌面、平板电脑和移动设备。它可以用于模拟特定设备的浏览器行为,例如用户代理、屏幕尺寸、视口以及是否启用触摸。所有测试都将使用指定的设备参数运行。
- 测试
- 库
import { defineConfig, devices } from '@playwright/test'; // import devices
export default defineConfig({
projects: [
{
name: 'chromium',
use: {
...devices['Desktop Chrome'],
},
},
{
name: 'Mobile Safari',
use: {
...devices['iPhone 13'],
},
},
],
});
const { chromium, devices } = require('playwright');
const browser = await chromium.launch();
const iphone13 = devices['iPhone 13'];
const context = await browser.newContext({
...iphone13,
});
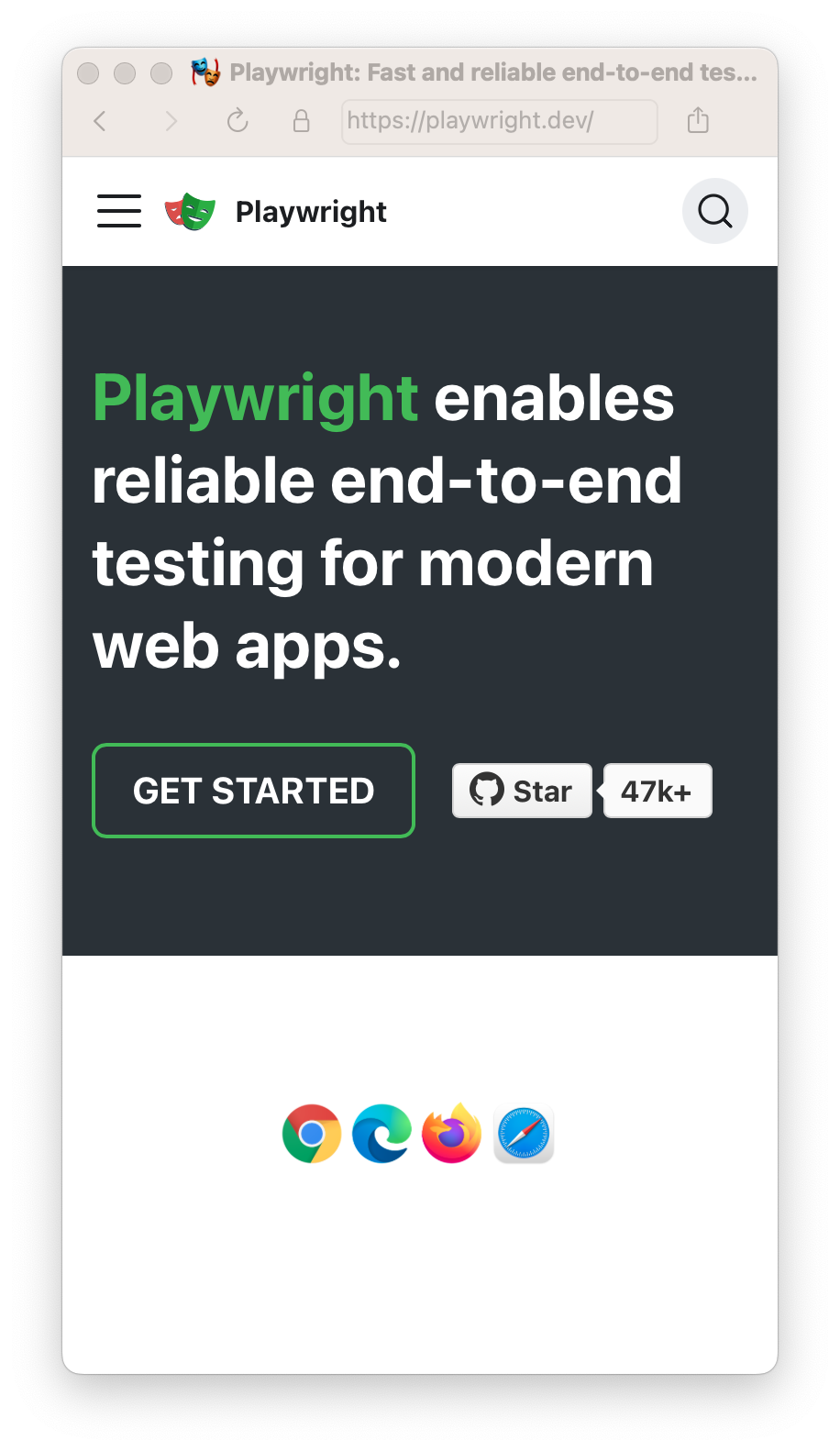
视口
视口包含在设备中,但您可以使用 page.setViewportSize() 为某些测试覆盖它。
- 测试
- 库
import { defineConfig, devices } from '@playwright/test';
export default defineConfig({
projects: [
{
name: 'chromium',
use: {
...devices['Desktop Chrome'],
// It is important to define the `viewport` property after destructuring `devices`,
// since devices also define the `viewport` for that device.
viewport: { width: 1280, height: 720 },
},
},
]
});
// Create context with given viewport
const context = await browser.newContext({
viewport: { width: 1280, height: 1024 }
});
测试文件
- 测试
- 库
import { test, expect } from '@playwright/test';
test.use({
viewport: { width: 1600, height: 1200 },
});
test('my test', async ({ page }) => {
// ...
});
// Create context with given viewport
const context = await browser.newContext({
viewport: { width: 1280, height: 1024 }
});
// Resize viewport for individual page
await page.setViewportSize({ width: 1600, height: 1200 });
// Emulate high-DPI
const context = await browser.newContext({
viewport: { width: 2560, height: 1440 },
deviceScaleFactor: 2,
});
同样适用于测试文件内部。
- 测试
- 库
import { test, expect } from '@playwright/test';
test.describe('specific viewport block', () => {
test.use({ viewport: { width: 1600, height: 1200 } });
test('my test', async ({ page }) => {
// ...
});
});
// Create context with given viewport
const context = await browser.newContext({
viewport: { width: 1600, height: 1200 }
});
const page = await context.newPage();
isMobile
是否考虑 meta 视口标签并启用触摸事件。
import { defineConfig, devices } from '@playwright/test';
export default defineConfig({
projects: [
{
name: 'chromium',
use: {
...devices['Desktop Chrome'],
// It is important to define the `isMobile` property after destructuring `devices`,
// since devices also define the `isMobile` for that device.
isMobile: false,
},
},
]
});
区域设置和时区
模拟用户区域设置和时区,可以在配置中为所有测试全局设置,然后为特定测试覆盖。
import { defineConfig } from '@playwright/test';
export default defineConfig({
use: {
// Emulates the user locale.
locale: 'en-GB',
// Emulates the user timezone.
timezoneId: 'Europe/Paris',
},
});
- 测试
- 库
import { test, expect } from '@playwright/test';
test.use({
locale: 'de-DE',
timezoneId: 'Europe/Berlin',
});
test('my test for de lang in Berlin timezone', async ({ page }) => {
await page.goto('https://www.bing.com');
// ...
});
const context = await browser.newContext({
locale: 'de-DE',
timezoneId: 'Europe/Berlin',
});
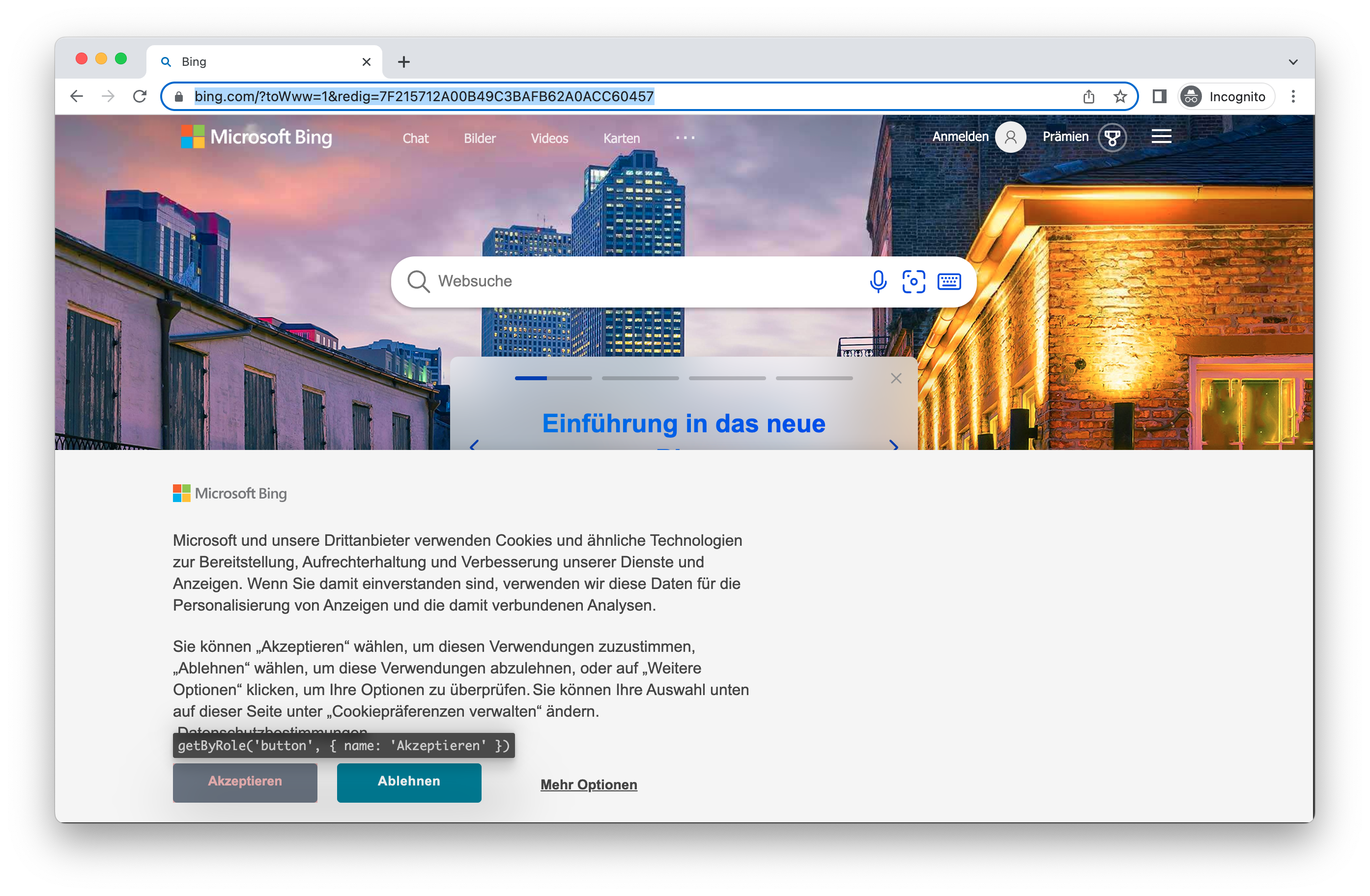
权限
允许应用程序显示系统通知。
- 测试
- 库
import { defineConfig } from '@playwright/test';
export default defineConfig({
use: {
// Grants specified permissions to the browser context.
permissions: ['notifications'],
},
});
const context = await browser.newContext({
permissions: ['notifications'],
});
允许特定域的通知。
- 测试
- 库
import { test } from '@playwright/test';
test.beforeEach(async ({ context }) => {
// Runs before each test and signs in each page.
await context.grantPermissions(['notifications'], { origin: 'https://skype.com' });
});
test('first', async ({ page }) => {
// page has notifications permission for https://skype.com.
});
await context.grantPermissions(['notifications'], { origin: 'https://skype.com' });
使用 browserContext.clearPermissions() 撤销所有权限。
// Library
await context.clearPermissions();
地理位置
授予 "geolocation"
权限并将地理位置设置为特定区域。
import { defineConfig } from '@playwright/test';
export default defineConfig({
use: {
// Context geolocation
geolocation: { longitude: 12.492507, latitude: 41.889938 },
permissions: ['geolocation'],
},
});
- 测试
- 库
import { test, expect } from '@playwright/test';
test.use({
geolocation: { longitude: 41.890221, latitude: 12.492348 },
permissions: ['geolocation'],
});
test('my test with geolocation', async ({ page }) => {
// ...
});
const context = await browser.newContext({
geolocation: { longitude: 41.890221, latitude: 12.492348 },
permissions: ['geolocation']
});
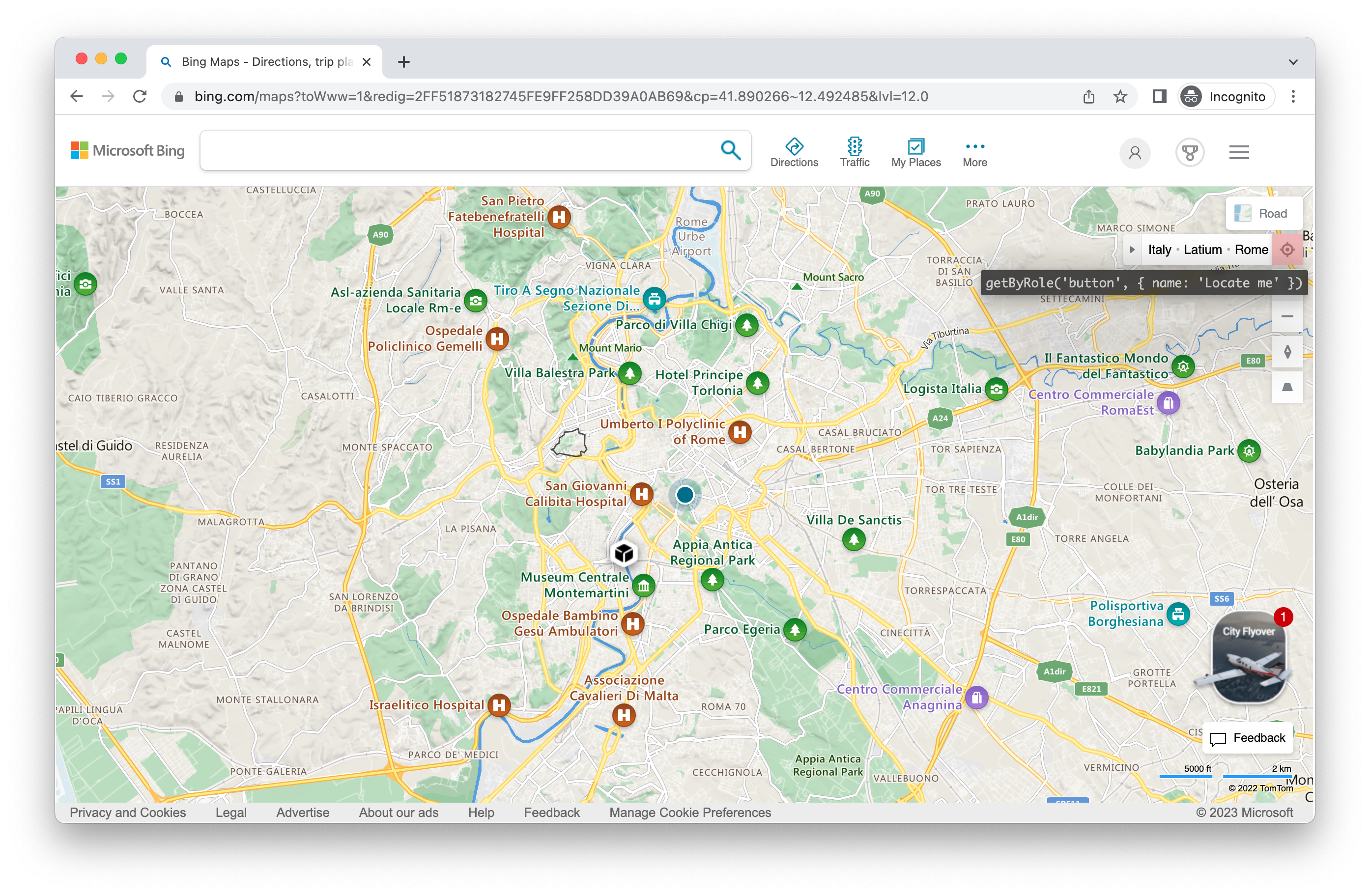
稍后更改位置
- 测试
- 库
import { test, expect } from '@playwright/test';
test.use({
geolocation: { longitude: 41.890221, latitude: 12.492348 },
permissions: ['geolocation'],
});
test('my test with geolocation', async ({ page, context }) => {
// overwrite the location for this test
await context.setGeolocation({ longitude: 48.858455, latitude: 2.294474 });
});
await context.setGeolocation({ longitude: 48.858455, latitude: 2.294474 });
注意您只能更改上下文中所有页面的地理位置。
颜色方案和媒体
模拟用户的 "colorScheme"
。支持的值为“light”和“dark”。您还可以使用 page.emulateMedia() 模拟媒体类型。
import { defineConfig } from '@playwright/test';
export default defineConfig({
use: {
colorScheme: 'dark',
},
});
- 测试
- 库
import { test, expect } from '@playwright/test';
test.use({
colorScheme: 'dark' // or 'light'
});
test('my test with dark mode', async ({ page }) => {
// ...
});
// Create context with dark mode
const context = await browser.newContext({
colorScheme: 'dark' // or 'light'
});
// Create page with dark mode
const page = await browser.newPage({
colorScheme: 'dark' // or 'light'
});
// Change color scheme for the page
await page.emulateMedia({ colorScheme: 'dark' });
// Change media for page
await page.emulateMedia({ media: 'print' });
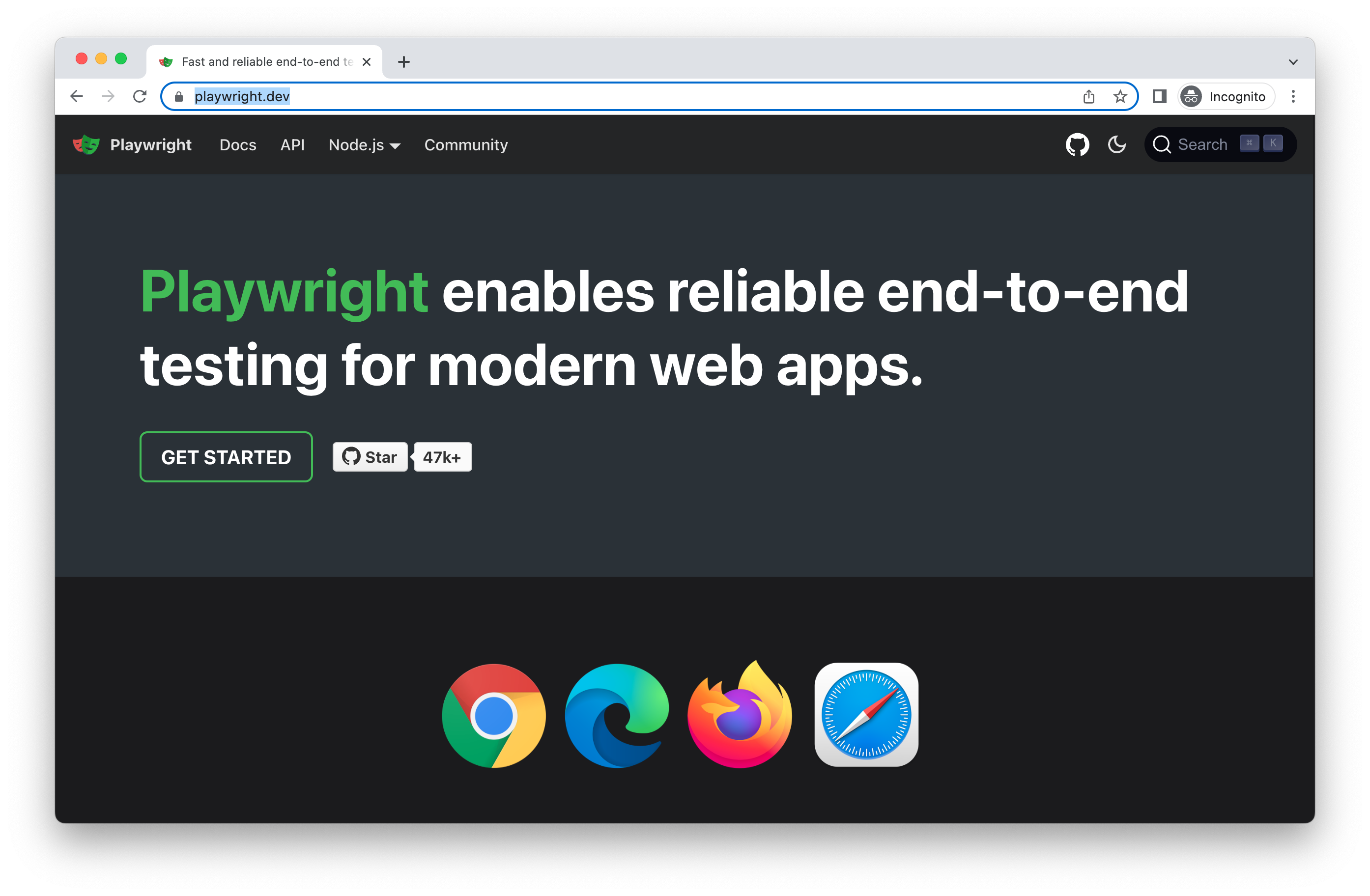
用户代理
用户代理包含在设备中,因此您很少需要更改它,但是如果您确实需要测试不同的用户代理,您可以使用 userAgent
属性覆盖它。
- 测试
- 库
import { test, expect } from '@playwright/test';
test.use({ userAgent: 'My user agent' });
test('my user agent test', async ({ page }) => {
// ...
});
const context = await browser.newContext({
userAgent: 'My user agent'
});
离线
模拟网络离线。
import { defineConfig } from '@playwright/test';
export default defineConfig({
use: {
offline: true
},
});
启用 JavaScript
模拟 JavaScript 被禁用的用户场景。
- 测试
- 库
import { test, expect } from '@playwright/test';
test.use({ javaScriptEnabled: false });
test('test with no JavaScript', async ({ page }) => {
// ...
});
const context = await browser.newContext({
javaScriptEnabled: false
});