项目
简介
项目是使用相同配置运行的测试的逻辑分组。我们使用项目以便可以在不同的浏览器和设备上运行测试。项目在 playwright.config.ts
文件中配置,配置完成后,您可以在所有项目或仅在特定项目上运行测试。您还可以使用项目在不同的配置中运行相同的测试。例如,您可以在登录和退出登录状态下运行相同的测试。
通过设置项目,您还可以使用不同的超时或重试次数运行一组测试,或者针对不同的环境(如 staging 和 production)运行一组测试,按 package/功能拆分测试等等。
为多个浏览器配置项目
通过使用 项目,您可以在多个浏览器(如 chromium、webkit 和 firefox)以及品牌浏览器(如 Google Chrome 和 Microsoft Edge)中运行测试。Playwright 也可以在模拟的平板电脑和移动设备上运行。请参阅设备参数注册表,获取精选的桌面、平板电脑和移动设备的完整列表。
import { defineConfig, devices } from '@playwright/test';
export default defineConfig({
projects: [
{
name: 'chromium',
use: { ...devices['Desktop Chrome'] },
},
{
name: 'firefox',
use: { ...devices['Desktop Firefox'] },
},
{
name: 'webkit',
use: { ...devices['Desktop Safari'] },
},
/* Test against mobile viewports. */
{
name: 'Mobile Chrome',
use: { ...devices['Pixel 5'] },
},
{
name: 'Mobile Safari',
use: { ...devices['iPhone 12'] },
},
/* Test against branded browsers. */
{
name: 'Microsoft Edge',
use: {
...devices['Desktop Edge'],
channel: 'msedge'
},
},
{
name: 'Google Chrome',
use: {
...devices['Desktop Chrome'],
channel: 'chrome'
},
},
],
});
运行项目
Playwright 默认将运行所有项目。
npx playwright test
Running 7 tests using 5 workers
✓ [chromium] › example.spec.ts:3:1 › basic test (2s)
✓ [firefox] › example.spec.ts:3:1 › basic test (2s)
✓ [webkit] › example.spec.ts:3:1 › basic test (2s)
✓ [Mobile Chrome] › example.spec.ts:3:1 › basic test (2s)
✓ [Mobile Safari] › example.spec.ts:3:1 › basic test (2s)
✓ [Microsoft Edge] › example.spec.ts:3:1 › basic test (2s)
✓ [Google Chrome] › example.spec.ts:3:1 › basic test (2s)
使用 --project
命令行选项来运行单个项目。
npx playwright test --project=firefox
Running 1 test using 1 worker
✓ [firefox] › example.spec.ts:3:1 › basic test (2s)
VS Code 测试运行器在默认浏览器 Chrome 上运行您的测试。要在其他/多个浏览器上运行,请单击测试侧边栏中播放按钮的下拉菜单,然后选择另一个配置文件,或者通过单击选择默认配置文件并选择您希望在其上运行测试的浏览器来修改默认配置文件。
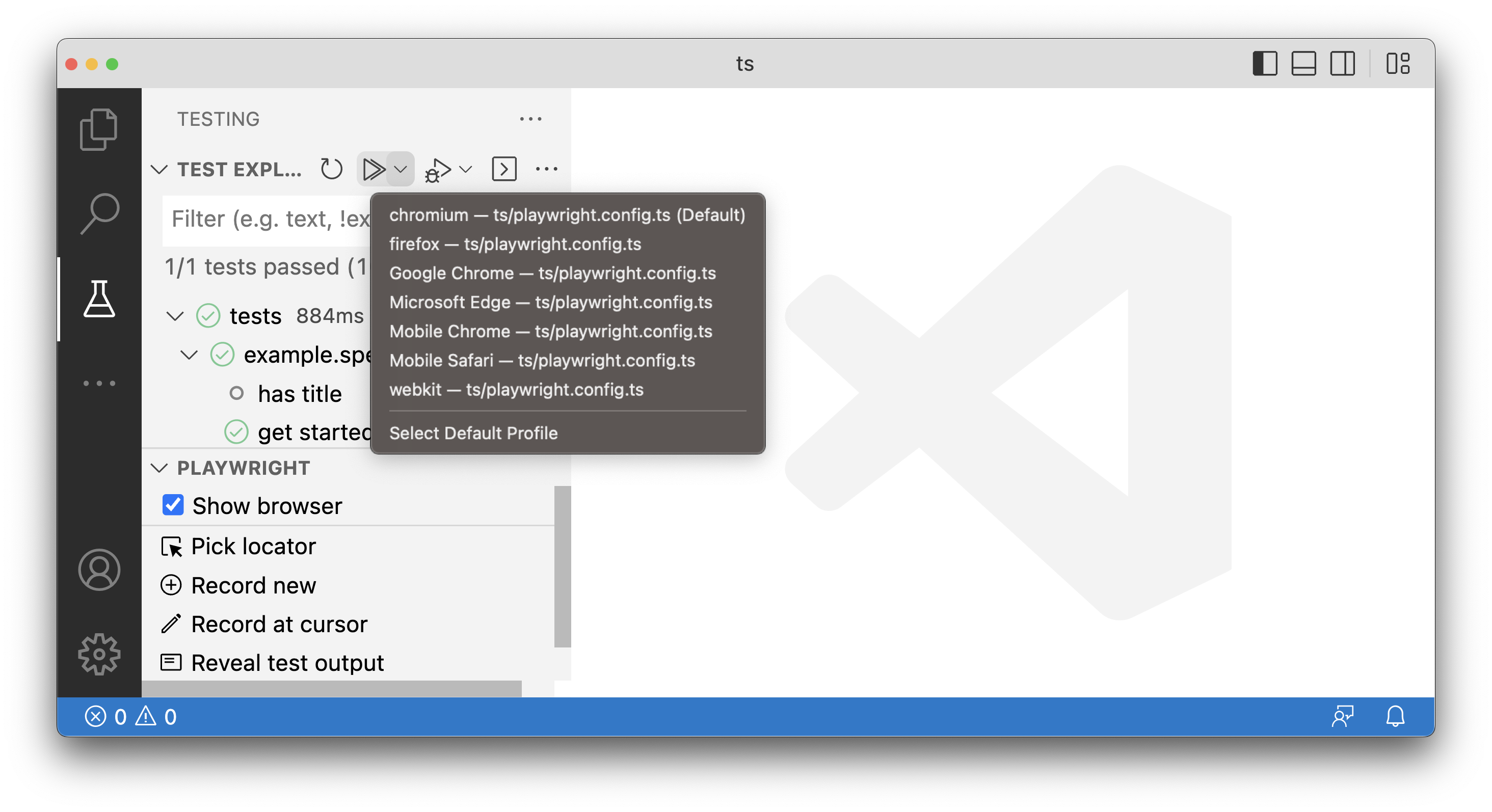
选择特定配置文件、各种配置文件或所有配置文件以运行测试。
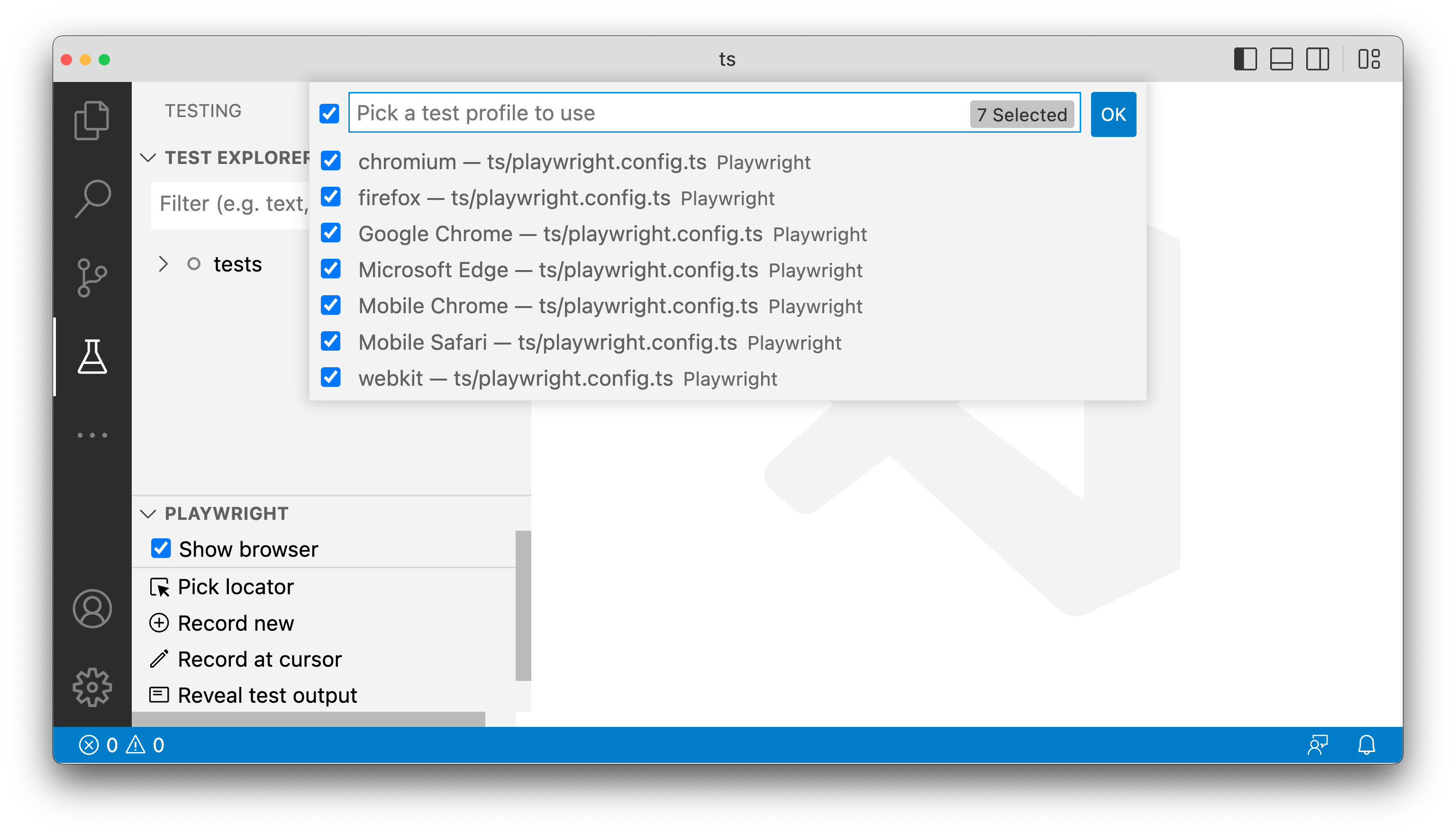
为多个环境配置项目
通过设置项目,我们还可以使用不同的超时或重试次数运行一组测试,或者针对不同的环境运行一组测试。例如,我们可以针对 staging 环境运行带有 2 次重试的测试,以及针对 production 环境运行带有 0 次重试的测试。
import { defineConfig } from '@playwright/test';
export default defineConfig({
timeout: 60000, // Timeout is shared between all tests.
projects: [
{
name: 'staging',
use: {
baseURL: 'staging.example.com',
},
retries: 2,
},
{
name: 'production',
use: {
baseURL: 'production.example.com',
},
retries: 0,
},
],
});
将测试拆分为项目
我们可以将测试拆分为项目,并使用过滤器来运行测试的子集。例如,我们可以创建一个项目,该项目使用过滤器运行与特定文件名匹配的所有测试。然后,我们可以有另一组测试来忽略特定的测试文件。
这是一个示例,它定义了一个通用超时和两个项目。“Smoke”项目运行一小部分没有重试的测试,“Default”项目运行所有其他带有重试的测试。
import { defineConfig } from '@playwright/test';
export default defineConfig({
timeout: 60000, // Timeout is shared between all tests.
projects: [
{
name: 'Smoke',
testMatch: /.*smoke.spec.ts/,
retries: 0,
},
{
name: 'Default',
testIgnore: /.*smoke.spec.ts/,
retries: 2,
},
],
});
依赖项
依赖项是需要在另一个项目中的测试运行之前运行的项目列表。它们对于配置全局 setup 操作非常有用,以便一个项目依赖于首先运行的项目。当使用项目依赖项时,测试报告器将显示 setup 测试,并且trace 查看器将记录 setup 的跟踪。您可以使用检查器来检查 setup 测试跟踪的 DOM 快照,并且您还可以在 setup 中使用fixtures。
在此示例中,chromium、firefox 和 webkit 项目依赖于 setup 项目。
import { defineConfig } from '@playwright/test';
export default defineConfig({
projects: [
{
name: 'setup',
testMatch: '**/*.setup.ts',
},
{
name: 'chromium',
use: { ...devices['Desktop Chrome'] },
dependencies: ['setup'],
},
{
name: 'firefox',
use: { ...devices['Desktop Firefox'] },
dependencies: ['setup'],
},
{
name: 'webkit',
use: { ...devices['Desktop Safari'] },
dependencies: ['setup'],
},
],
});
运行顺序
当使用具有依赖项的测试时,依赖项将始终首先运行,并且一旦此项目中的所有测试都通过,则其他项目将并行运行。
运行顺序
-
“setup”项目中的测试运行。一旦此项目中的所有测试都通过,则依赖项目中的测试将开始运行。
-
“chromium”、“webkit”和“firefox”项目中的测试一起运行。默认情况下,这些项目将并行运行,但受最大工作进程数的限制。
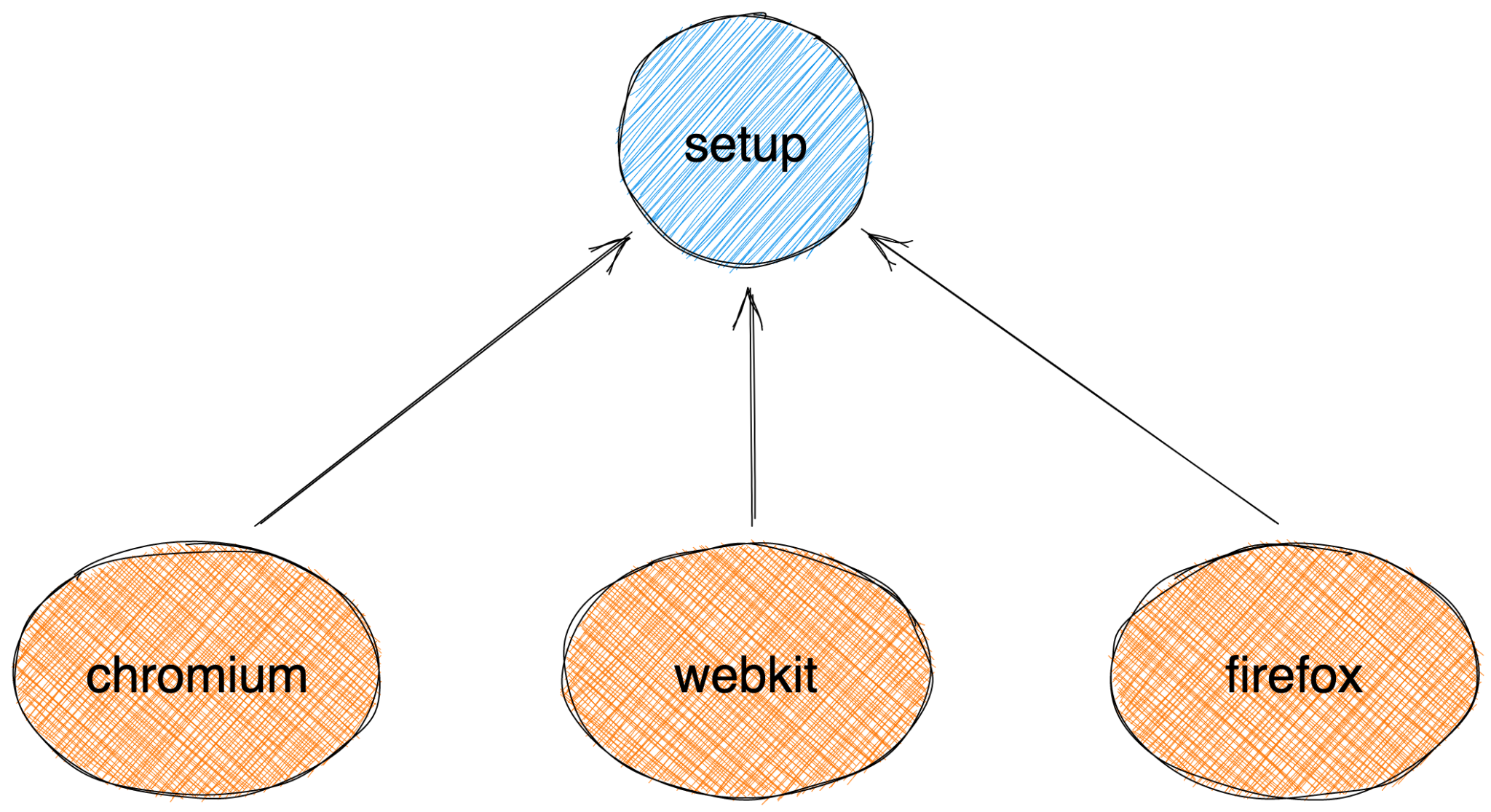
如果存在多个依赖项,则这些项目依赖项将首先并行运行。如果来自依赖项的测试失败,则依赖于此项目的测试将不会运行。
运行顺序
- “Browser Login”和“DataBase”项目中的测试并行运行
- “Browser Login” 通过
- ❌ “DataBase” 失败!
- “e2e tests” 项目未运行!
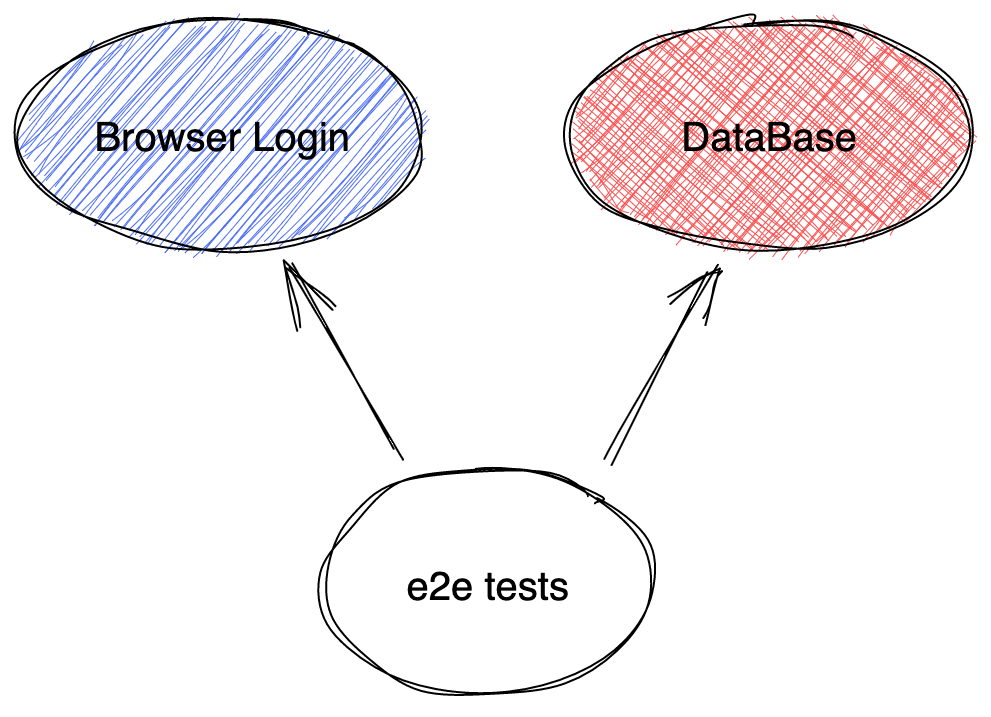
Teardown
您还可以通过向 setup 项目添加 testProject.teardown 属性来 teardown 您的 setup。Teardown 将在所有依赖项目运行后运行。请参阅 teardown 指南 以获取更多信息。
测试过滤
如果使用 --grep/--grep-invert
或 --shard
选项,则在 命令行 或 test.only() 中指定了测试文件名过滤器,它将仅应用于项目依赖链中最深层项目中的测试。换句话说,如果匹配的测试属于具有项目依赖项的项目,则 Playwright 将运行项目依赖项中的所有测试,而忽略过滤器。
自定义项目参数
项目还可用于使用您的自定义配置参数化测试 - 请查看此单独的指南。